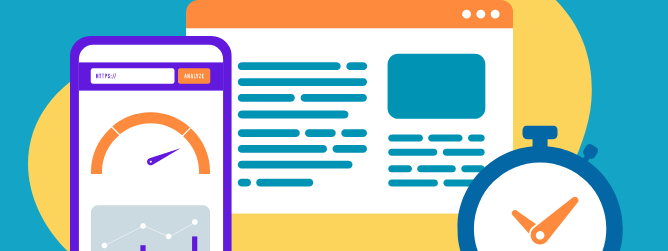
This is part two of our look at our work with Phreesia creating a custom WordPress theme. If you haven’t already, be kind, rewind, and take a look at part one. To summarize, we were challenged with building a custom WordPress theme that would grow with Phreesia, rather than hamper their growth as their prior theme was doing. As a company offering software solutions for patients and care providers, it was critical that Phreesia’s own site be both user-friendly and fast.
Code Bloat
The main culprit causing Phreesia’s slow site speeds were a number of plug-ins and third-party apps, added to provide quick solutions and front-end formatting. But each of these came with a price: layers upon layers of code, eventually taking a massive toll on load times.
To resolve this, we started a build from scratch and only brought in the minimum number of plug-ins and tools to ensure the site remained reliable, sleek, scalable, and speedy. For each task, we kept these values top-of-mind, ensuring we only included the necessary and intentional — while still setting them up for future success. We used four plug-ins and tools to accomplish this feat: Bootstrap, Advanced Custom Fields, FacetWP, and GenerateBlocks.
Bootstrap
First we began with Bootstrap, one of our favorite front-end development tools to quickly and easily create a number of elements. We went on to customize these elements to give Phreesia a unique yet stable and scalable design.
// functions.php
<?php
if ( ! defined( 'PHR_VERSION' ) ) {
// Replace the version number of the theme on each release.
define( 'PHR_VERSION', '1.0.0' );
}
if ( ! function_exists( 'phr_scripts' ) ) :
/**
* Enqueue scripts and styles.
*/
function phr_scripts() {
wp_enqueue_style( 'phr-style', get_stylesheet_uri(), array(), PHR_VERSION );
wp_style_add_data( 'phr-style', 'rtl', 'replace' );
}
endif;
add_action( 'wp_enqueue_scripts', 'phr_scripts' );
// style.scss
// 1. Include functions first (so you can manipulate colors, SVGs, calc, etc)
@import "../node_modules/bootstrap/scss/functions";
// 2. Include any default variable overrides here
// 3. Include remainder of required Bootstrap stylesheets (including any separate color mode stylesheets)
@import "../node_modules/bootstrap/scss/variables";
@import "../node_modules/bootstrap/scss/variables-dark";
// 4. Include any default map overrides here
// 5. Include remainder of required parts
@import "../node_modules/bootstrap/scss/maps";
@import "../node_modules/bootstrap/scss/mixins";
@import "../node_modules/bootstrap/scss/root";
// 6. Optionally include any other parts as needed
@import "../node_modules/bootstrap/scss/utilities";
@import "../node_modules/bootstrap/scss/reboot";
@import "../node_modules/bootstrap/scss/type";
@import "../node_modules/bootstrap/scss/images";
@import "../node_modules/bootstrap/scss/containers";
@import "../node_modules/bootstrap/scss/grid";
@import "../node_modules/bootstrap/scss/helpers";
// 7. Optionally include utilities API last to generate classes based on the Sass map in `_utilities.scss`
@import "../node_modules/bootstrap/scss/utilities/api";
// 8. Add additional custom code here
The first snippet is for loading the CSS stylesheet, while the second snippet is for loading the bootstrap library as explained here.
Advanced Custom Fields
The Advanced Custom Fields plug-in (ACF) was selected to help us create reusable blocks within WordPress’ Gutenberg block editor. This allowed us to add dynamic and interactive elements to the website, such as testimonials, pricing plans, contact forms, and more while maintaining their WYSIWYG (“What You See Is What You Get”) editing capabilities. We also used this tool to create custom fields for each user profile, such as location, role, and preferences.
// group_5ac3a0282aa9b.json
{
"key": "group_5ac3a0282aa9b",
"title": "Phreesia Options",
"fields": [
{
"key": "field_589de14dd984c",
"label": "Featured Blog Post",
"name": "featured_blog_post",
"type": "radio",
"instructions": "",
"required": 1,
"conditional_logic": 0,
"wrapper": {
"width": "",
"class": "",
"id": ""
},
"choices": {
"Yes": "Yes",
"No": "No"
},
"allow_null": 0,
"other_choice": 0,
"default_value": "No",
"layout": "vertical",
"return_format": "value",
"save_other_choice": 0
},
{
"key": "field_630e60bb51e33",
"label": "Whitepaper URL",
"name": "whitepaper_url",
"type": "url",
"instructions": "Add link toPDF here - download icon will automatically appear in share component and download button will be added to bottom of page",
"required": 0,
"conditional_logic": 0,
"wrapper": {
"width": "",
"class": "",
"id": ""
},
"default_value": "",
"placeholder": ""
},
{
"key": "field_630e60d351e34",
"label": "Download Button Label",
"name": "download_button_label",
"type": "text",
"instructions": "Update Download button label if needed",
"required": 0,
"conditional_logic": [
[
{
"field": "field_630e60bb51e33",
"operator": "!=empty"
}
]
],
"wrapper": {
"width": "",
"class": "",
"id": ""
},
"default_value": "Download the full whitepaper",
"placeholder": "",
"prepend": "",
"append": "",
"maxlength": ""
},
{
"key": "field_6324c10c3e1f3",
"label": "Show Button?",
"name": "show_button",
"type": "radio",
"instructions": "Use this if you want to hide the download button but still have the download icon in the share component",
"required": 0,
"conditional_logic": [
[
{
"field": "field_630e60bb51e33",
"operator": "!=empty"
}
]
],
"wrapper": {
"width": "",
"class": "",
"id": ""
},
"choices": {
"Yes": "Yes",
"No": "No"
},
"allow_null": 0,
"other_choice": 0,
"default_value": "Yes",
"layout": "horizontal",
"return_format": "value",
"save_other_choice": 0
}
],
"location": [
[
{
"param": "post_type",
"operator": "==",
"value": "post"
},
{
"param": "post_category",
"operator": "==",
"value": "category:blog"
}
],
[
{
"param": "post_type",
"operator": "==",
"value": "post"
},
{
"param": "post_category",
"operator": "==",
"value": "category:insights"
}
],
[
{
"param": "post_type",
"operator": "==",
"value": "post"
},
{
"param": "post_category",
"operator": "==",
"value": "category:press-releases"
}
],
[
{
"param": "post_type",
"operator": "==",
"value": "post"
},
{
"param": "post_category",
"operator": "==",
"value": "category:webinars-events"
}
]
],
"menu_order": 0,
"position": "side",
"style": "default",
"label_placement": "top",
"instruction_placement": "label",
"hide_on_screen": "",
"active": true,
"description": "",
"show_in_rest": 0,
"modified": 1667243579
}
The ACF plugin automatically generates this code, which is simply a set of rules based on the fields we define. This file should be stored under the acf-json directory as explained here.
FacetWP
Another tool we used was FacetWP, which let us pull custom and dynamic database information into Gutenberg pages. This enabled us to display relevant and personalized content on the website, such as case studies, blog posts, events, and more. We also used this tool to create custom taxonomies for each category of content, such as software solutions, industry sectors, and customer stories, creating a number of different filters that could be applied.
<div class="card-list">
<?php while ( have_posts() ) : the_post(); ?>
<div class="card">
<?php the_post_thumbnail(); ?>
<div class="card-body">
<div class="card-text">
<?php the_date(); ?>
</div><!-- .card-text -->
<a
class="card-link"
href="<?php echo esc_url( get_permalink() ); ?>"
rel="bookmark"
>
<?php the_title( '<h2 class="card-title">', '</h2>' ); ?>
</a><!-- .card-link -->
</div><!-- .card-body -->
</div><!-- .card -->
<?php endwhile; wp_reset_postdata(); ?>
</div><!-- .card-list -->
Configured in the FacetWP settings, this block loops the blog listing page.
GenerateBlocks
Additionally, we used GenerateBlocks to help create custom landing pages for specific tasks like ad campaigns. This allowed us to create landing pages that were optimized for conversions and performance, such as lead generation forms, product demos, free trials, and more. We also used this tool to create custom post types that could be used within Gutenberg.
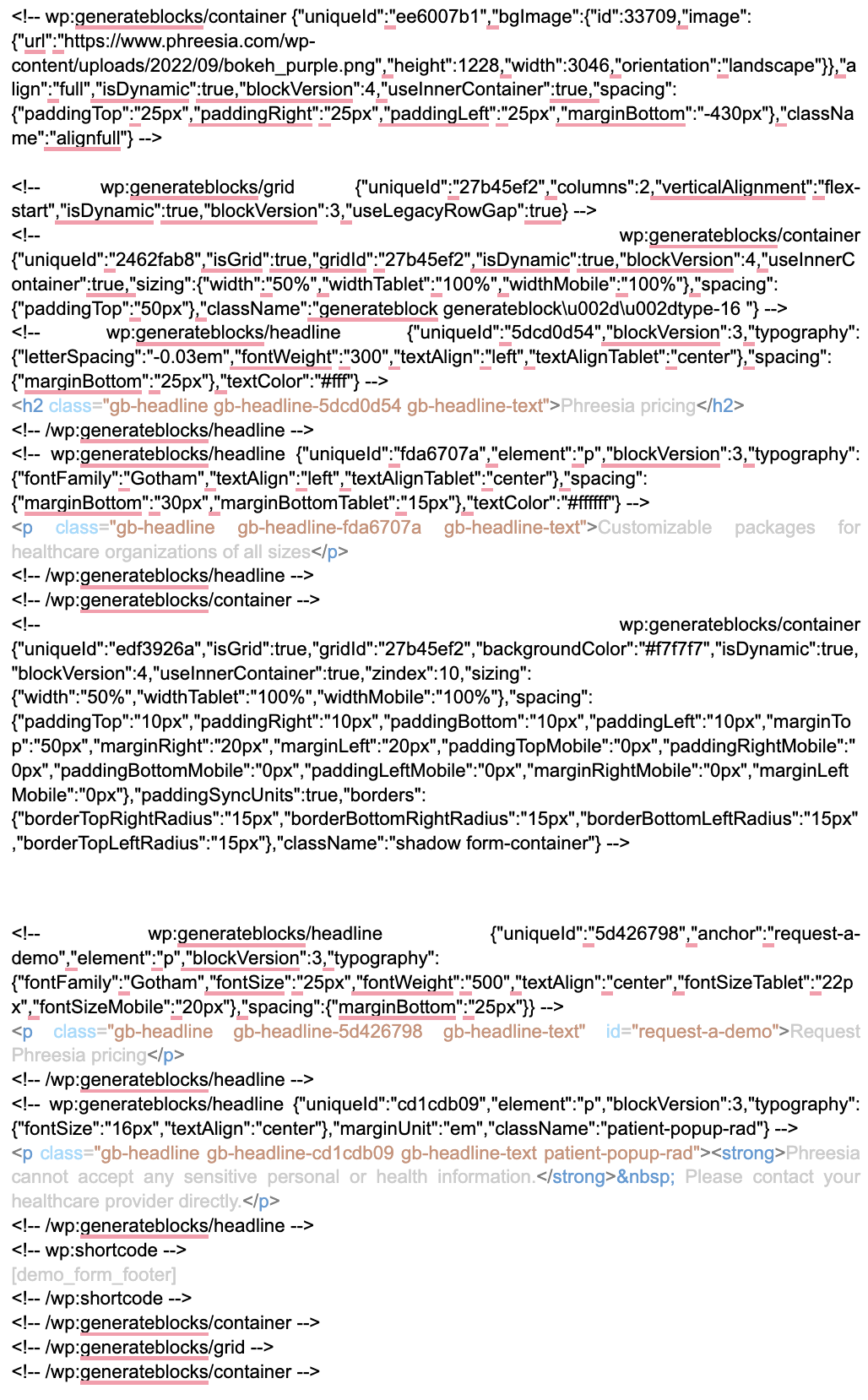
<!-- wp:generateblocks/container {"uniqueId":"ee6007b1","bgImage":{"id":33709,"image":{"url":"https://www.phreesia.com/wp-content/uploads/2022/09/bokeh_purple.png", "height":1228,"width":3046,"orientation":"landscape"}},"align":"full", "isDynamic":true,"blockVersion":4,"useInnerContainer":true,"spacing":{"paddingTop":"25px","paddingRight":"25px","paddingLeft":"25px", "marginBottom":"-430px"},"className":"alignfull"} -->
<!-- wp:generateblocks/grid {"uniqueId":"27b45ef2","columns":2,"verticalAlignment":"flex-start","isDynamic":true,"blockVersion":3,"useLegacyRowGap":true} -->
<!-- wp:generateblocks/container {"uniqueId":"2462fab8","isGrid":true,"gridId":"27b45ef2","isDynamic":true, "blockVersion":4,"useInnerContainer":true,"sizing":{"width":"50%","widthTablet":"100%","widthMobile":"100%"}, "spacing":{"paddingTop":"50px"},"className":"generateblock generateblock\u002d\u002dtype-16 "} -->
<!-- wp:generateblocks/headline {"uniqueId":"5dcd0d54","blockVersion":3,"typography":{"letterSpacing":"-0.03em","fontWeight":"300","textAlign":"left","textAlignTablet":"center"},"spacing":{"marginBottom":"25px"},"textColor":"#fff"} -->
<h2 class="gb-headline gb-headline-5dcd0d54 gb-headline-text">Phreesia pricing</h2>
<!-- /wp:generateblocks/headline -->
<!-- wp:generateblocks/headline {"uniqueId":"fda6707a","element":"p","blockVersion":3,"typography":{"fontFamily":"Gotham","textAlign":"left","textAlignTablet":"center"},"spacing":{"marginBottom":"30px","marginBottomTablet":"15px"},"textColor":"#ffffff"} -->
<p class="gb-headline gb-headline-fda6707a gb-headline-text">Customizable packages for healthcare organizations of all sizes</p>
<!-- /wp:generateblocks/headline -->
<!-- /wp:generateblocks/container -->
<!-- wp:generateblocks/container {"uniqueId":"edf3926a","isGrid":true, "gridId":"27b45ef2","backgroundColor":"#f7f7f7","isDynamic":true,"blockVersion":4, "useInnerContainer":true,"zindex":10,"sizing":{"width":"50%","widthTablet":"100%", "widthMobile":"100%"},"spacing":{"paddingTop":"10px","paddingRight":"10px", "paddingBottom":"10px","paddingLeft":"10px","marginTop":"50px", "marginRight":"20px","marginLeft":"20px","paddingTopMobile":"0px", "paddingRightMobile":"0px","paddingBottomMobile":"0px", "paddingLeftMobile":"0px","marginRightMobile":"0px","marginLeftMobile":"0px"}, "paddingSyncUnits":true,"borders":{"borderTopRightRadius":"15px", "borderBottomRightRadius":"15px","borderBottomLeftRadius":"15px", "borderTopLeftRadius":"15px"},"className":"shadow form-container"} -->
<!-- wp:generateblocks/headline {"uniqueId":"5d426798","anchor":"request-a-demo","element":"p","blockVersion":3,"typography":{"fontFamily":"Gotham","fontSize":"25px","fontWeight":"500", "textAlign":"center","fontSizeTablet":"22px","fontSizeMobile":"20px"}, "spacing":{"marginBottom":"25px"}} -->
<p class="gb-headline gb-headline-5d426798 gb-headline-text" id="request-a-demo">Request Phreesia pricing</p>
<!-- /wp:generateblocks/headline -->
<!-- wp:generateblocks/headline {"uniqueId":"cd1cdb09","element":"p","blockVersion":3,"typography":{"fontSize":"16px","textAlign":"center"},"marginUnit":"em","className":"patient-popup-rad"} -->
<p class="gb-headline gb-headline-cd1cdb09 gb-headline-text patient-popup-rad"><strong>Phreesia cannot accept any sensitive personal or health information.</strong> Please contact your healthcare provider directly.</p>
<!-- /wp:generateblocks/headline -->
<!-- wp:shortcode -->
[demo_form_footer]
<!-- /wp:shortcode -->
<!-- /wp:generateblocks/container -->
<!-- /wp:generateblocks/grid -->
<!-- /wp:generateblocks/container -->
The Gutenberg editor markup above demonstrates how to use the container, grid, and headline blocks.
More From Our Team
With these tools and our combined expertise in web development, we were able to create a truly one-of-a-kind site for Phreesia that met their high standards and expectations while improving loading times by up to 80% from their prior site. This will allow Phreesia to continue to wow their customers with a speedy, scalable site that will serve them for years to come.